CDS Demo: Using Climate Data Store API
Contents
CDS Demo: Using Climate Data Store API#
The Copernicus Climate Data Store API is a Python library to download data from the Climate Data Store.
https://pypi.org/project/cdsapi/
You can install it using conda
:
conda install -c conda-forge cdsapi
You need to add your api key into ~/.cdsapirc
.
Init CDSAPI#
import cdsapi
c = cdsapi.Client()
Download CMIP6 data#
c.retrieve(
'projections-cmip6',
{
'temporal_resolution': 'monthly',
'experiment': 'historical',
'level': 'single_levels',
'variable': 'near_surface_air_temperature',
'model': 'mpi_esm1_2_hr',
'date': '2000-01-01/2000-01-31',
'area': [
70, -40, -40,
70,
],
'format': 'zip',
},
'download.zip')
/home/k/k204199/.conda/envs/summerschool_2022/lib/python3.10/site-packages/urllib3/connectionpool.py:1045: InsecureRequestWarning: Unverified HTTPS request is being made to host 'cds.climate.copernicus.eu'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/1.26.x/advanced-usage.html#ssl-warnings
warnings.warn(
2022-08-31 09:58:14,962 INFO Welcome to the CDS
2022-08-31 09:58:14,963 INFO Sending request to https://cds.climate.copernicus.eu/api/v2/resources/projections-cmip6
/home/k/k204199/.conda/envs/summerschool_2022/lib/python3.10/site-packages/urllib3/connectionpool.py:1045: InsecureRequestWarning: Unverified HTTPS request is being made to host 'cds.climate.copernicus.eu'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/1.26.x/advanced-usage.html#ssl-warnings
warnings.warn(
2022-08-31 09:58:15,064 INFO Request is completed
2022-08-31 09:58:15,064 INFO Downloading https://download-0021.copernicus-climate.eu/cache-compute-0021/cache/data6/adaptor.esgf_wps.retrieve-1661764688.1551287-13046-20-50e09f56-f2e3-4f3f-b7fe-abca68c05989.zip to download.zip (204.5K)
/home/k/k204199/.conda/envs/summerschool_2022/lib/python3.10/site-packages/urllib3/connectionpool.py:1045: InsecureRequestWarning: Unverified HTTPS request is being made to host 'download-0021.copernicus-climate.eu'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/1.26.x/advanced-usage.html#ssl-warnings
warnings.warn(
0%| | 0.00/204k [00:00<?, ?B/s]
2022-08-31 09:58:15,404 INFO Download rate 602.4K/s
Result(content_length=209385,content_type=application/zip,location=https://download-0021.copernicus-climate.eu/cache-compute-0021/cache/data6/adaptor.esgf_wps.retrieve-1661764688.1551287-13046-20-50e09f56-f2e3-4f3f-b7fe-abca68c05989.zip)
Use command line to extract downloaded data#
!unzip -o download.zip
Archive: download.zip
extracting: tas_Amon_MPI-ESM1-2-HR_historical_r1i1p1f1_gn_20000116-20000116_v20190710.nc
extracting: adaptor.esgf_wps.retrieve-1661764688.1551287-13046-20-50e09f56-f2e3-4f3f-b7fe-abca68c05989_provenance.json
extracting: adaptor.esgf_wps.retrieve-1661764688.1551287-13046-20-50e09f56-f2e3-4f3f-b7fe-abca68c05989_provenance.png
Use xarray to open CMIP6 data#
import xarray as xr
ds = xr.open_dataset("tas_Amon_MPI-ESM1-2-HR_historical_r1i1p1f1_gn_20000116-20000116_v20190710.nc")
ds
<xarray.Dataset> Dimensions: (time: 1, bnds: 2, lat: 118, lon: 117) Coordinates: * time (time) datetime64[ns] 2000-01-16T12:00:00 * lat (lat) float64 -39.74 -38.81 -37.87 -36.93 ... 67.79 68.73 69.66 * lon (lon) float64 -39.38 -38.44 -37.5 -36.56 ... 67.5 68.44 69.38 height float64 2.0 Dimensions without coordinates: bnds Data variables: time_bnds (time, bnds) datetime64[ns] 2000-01-01 2000-02-01 lat_bnds (lat, bnds) float64 -40.21 -39.27 -39.27 ... 69.19 69.19 70.13 lon_bnds (lon, bnds) float64 -39.84 -38.91 -38.91 ... 68.91 68.91 69.84 tas (time, lat, lon) float32 289.4 289.2 288.9 ... 256.7 256.1 255.4 Attributes: (12/47) Conventions: CF-1.7 CMIP-6.2 activity_id: CMIP branch_method: standard branch_time_in_child: 0.0 branch_time_in_parent: 0.0 contact: cmip6-mpi-esm@dkrz.de ... ... title: MPI-ESM1-2-HR output prepared for CMIP6 variable_id: tas variant_label: r1i1p1f1 license: CMIP6 model data produced by MPI-M is licensed un... cmor_version: 3.5.0 tracking_id: hdl:21.14100/af75dd9f-d9c2-4e0e-a294-2bb0d5b740cf
Plot CMIP6 data#
ds.tas.isel(time=0).plot()
<matplotlib.collections.QuadMesh at 0x7fff4f6122c0>
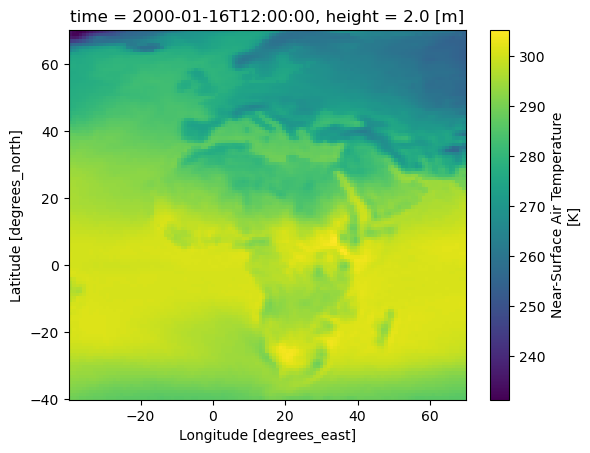